【Unityの勉強中】Unity5の参考書がUnityScriptの本だったので、C#に四苦八苦で自前書き換え
現在、参考書を片手にUnityの勉強中なのですが、
その過程でJavaScriptのコードををC#に置き換える必要があって、四苦八苦だったので、今回はそのお話です。
参考書はUnityScript(JavaScript)だった…
今読んでいるUnity5の参考書『Unity5入門 最新開発環境による簡単3D&2Dゲーム制作』ですが…
5章の学習を進めていたら、使っているコードがUnityScript(JavaScript)で、そのままでは全く動きませんでした。
(これから買う方は、Unity2018入門の本があるので、そちらを買いましょうね)
サンプルで用意されていたキャラクタを取り込んだけど、操作させる事ができなかったんです。
でも、その時は、Unity5と2018との違いで上手く動かないのかな?くらいの感じでした。
(追跡カメラもデフォルトで追加できていませんでしたから)
ですが、5章で実際にコードを扱う所で気づきました…「これC#じゃない!!」
どうやら、この書籍。UnityScript(JavaScript)で書かれている書籍だったみたいです。
……改めて"はじめに"とか読み返したけど、そんな記述どこにも書かれてなかった…
学習を進めてたら、いきなりJavaScriptが出てきてびっくりですよ。
こう言うことは、ちゃんと書いておいて欲しいです。
もうUnityScript(JavaScript)はNG?
全く動かないのでは、そこから先の学習に支障をきたすので、何とか動かそうと試みたのですが…
どうやら、最新バージョンの「2018.4.14f1」(2020/2/10時点)では動かせないみたいです。
***.jsはソースファイルとして認識してくれないし、オブジェクトに適用する事もできない…
仕方がないので、何とか簡単にC#に変換する方法がないか調べたのですけど…
『unityscript2csharp』 と言うツールがあるみたいですが、使い方がよく分からなかったです。
Windows環境なら、*.exeの実行ファイルで動かせるみたいなんですけどね…Macでは動かせないんでしょうか?
と言うわけで、UnityScriptをそのまま活用するのは諦めることにしました。
こうなったら自前でC#で書き直す方が早い!
まぁ、そんなこんなで色々と試行錯誤するなら、自前でC#に置き換えた方が早い!
と言うわけで、乏しい知識の中でGoogle先生のお世話になりながら、頑張って書き換えました。
せっかくなので、何かの参考になるかも知れないので、その書き換え前後のコードを公開しておきます。
Chapter5-03「TimerCount.js」
UnityScript(JavaScript)コード「TimerCount.js」
#pragma strict import UnityEngine.UI; private var timerText : Text; private var time : float; private var currentTime : int; public static var stop : boolean = false; function Start () { timerText = GetComponent.(); } function Update () { if(stop){ time += Time.deltaTime; currentTime = time; timerText.text = "Timer :" + currentTime; } }
C#コード「TimerCount.cs」
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class TimerCount : MonoBehaviour { private Text timerText; private float time; private int currentTime; static public bool stop = false; // Start is called before the first frame update void Start() { timerText = GetComponent (); } // Update is called once per frame void Update() { if (stop) { time += Time.deltaTime; currentTime = Mathf.CeilToInt(time); timerText.text = "Timer:" + currentTime.ToString("D3"); } } }
Chapter5-03「Click.js」
UnityScript(JavaScript)コード「Click.js」
#pragma strict public function StopTimer(){ if(TimerCount.stop){ TimerCount.stop = false; }else{ TimerCount.stop = true; } }
C#コード「Click.cs」
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Click : MonoBehaviour { // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { } public void StopTimer() { if (TimerCount.stop) { TimerCount.stop = false; } else { TimerCount.stop = true; } } }
(おまけ)PQchan「QueryChanController.js」
Chapter5で扱うのは、今までの2つのコードだけなのですが、
実はChapter4で取り込んだPQchanでもUnityScriptが使われていましたので、それも書き換えました。
UnityScript(JavaScript)コード「QueryChanController.js」
#pragma strict public var speed : float = 3.0f; public var jumpPower : float = 6.0f; private var direction : Vector3; private var controller : CharacterController; private var anim : Animator; function Start () { controller = GetComponent.(); anim = GetComponent.(); } function Update () { if(controller.isGrounded){ var inputX : float = Input.GetAxis("Horizontal"); var inputY : float = Input.GetAxis("Vertical"); var inputDirection : Vector3 = Vector3(inputX,0,inputY); direction = Vector3.zero; if(inputDirection.magnitude > 0.1f){ transform.LookAt(transform.position + inputDirection); direction += transform.forward * speed; anim.SetFloat("Speed",direction.magnitude); }else{ anim.SetFloat("Speed",0); } if(Input.GetButton("Jump")){ anim.SetTrigger("Jump"); direction.y += jumpPower; } } controller.Move(direction * Time.deltaTime); direction.y += Physics.gravity.y * Time.deltaTime; }
C#コード「QueryChanController.cs」
using System.Collections; using System.Collections.Generic; using UnityEngine; public class QueryChanController : MonoBehaviour { public float speed = 3.0f; public float jumpPower = 6.0f; private Vector3 direction; private CharacterController controller; private Animator anim; // Start is called before the first frame update void Start() { controller = GetComponent(); anim = GetComponent(); } // Update is called once per frame void Update() { if (controller.isGrounded) { float inputX = Input.GetAxis("Horizontal"); float inputY = Input.GetAxis("Vertical"); Vector3 inputDirection = new Vector3(inputX,0,inputY); direction = Vector3.zero; if (inputDirection.magnitude > 0.1f) { transform.LookAt(transform.position + inputDirection); direction += transform.forward * speed; anim.SetFloat("Speed", direction.magnitude); } else { anim.SetFloat("Speed", 0); } if (Input.GetButton("Jump")) { anim.SetTrigger("Jump"); direction.y += jumpPower; } } controller.Move(direction * Time.deltaTime); direction.y += Physics.gravity.y * Time.deltaTime; } }
このコードをPQchanに適用する事で、ちゃんとクエリちゃんを操作できる様になりました。
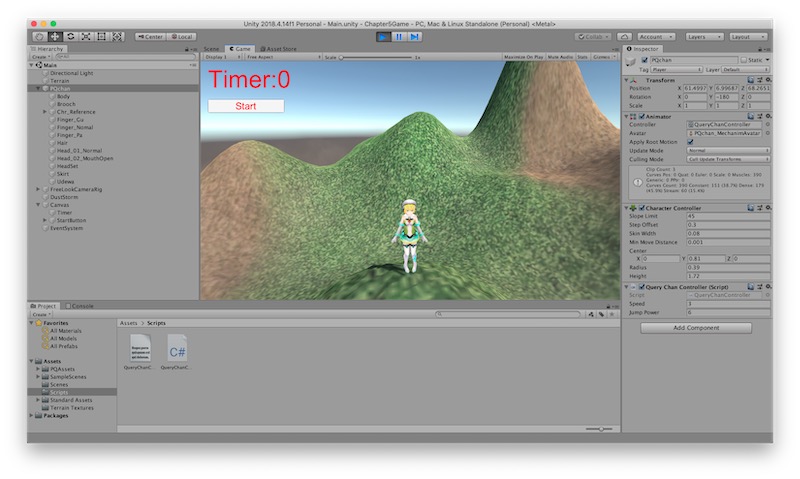
残り3章も…
一応、今回の自前書き換えで、Chapter5は何とか乗り切る事ができました。
書き換えたコードで思った通りの動作をしているので、問題はないと思います。
ですが、この参考書…Chapter8まであるので、後3章も残ってます…
…後、どれくらいコードを書く必要があるのでしょう?
でも、可能な限り、残りのコードも書き換えて公開できればと思います。
とりあえず、この本を最後まで終わらせるぞぉ〜!
ディスカッション
コメント一覧
まだ、コメントがありません